What's an email API?
An email API (Application Programmable Interface) allows developers to access email functionalities for their applications. With an email API, users can connect an application or service to an email service provider and use the email functionalities for their service.
How does an email API work?
An email API is an intermediary between an application or service and the email service provider's server.
How do you choose the right email API?
Email API falls broadly into three categories—business emails, transactional emails, and contextual emails. You can choose the email API that suits your needs the best.
Business email API
Email APIs that are provided by your business email provider. These APIs are predominantly used to create integrations for your email inbox. Zoho Mail provides a robust set of business email APIs.
Transactional email API
These email APIs handle the automated emails triggered by a user action on your application or site, such as OTP emails or invoice emails, for example. ZeptoMail is a reliable and secure transactional email API provider.
Contextual email API
Contextual email APIs are designed to provide full email capabilities, essentially acting as an email engine to build your application on. Zoho Mail360 is an email API platform that can power the email workflows in your application.
Transactional email APIs
Integrate your application to ZeptoMail in minutes using our email APIs to send out transactional or notification emails. Some of the most common email API use cases are:
- Order confirmations
- Shipping tracking
- OTP emails
- Verification emails
- Account activity alert
- Security alerts
- Billing and invoice alerts
- Order confirmations
- Shipping tracking
- OTP emails
- Verification emails
- Account activity alert
- Security alerts
- Billing and invoice alerts
Developer-friendly transactional email APIs
Experience hassle-free integration with ZeptoMail's email APIs using our code libraries in the programming language of your choice.
curl "https://zeptomail.zoho.com/v1.1/email" \
-X POST \
-H "Accept: application/json" \
-H "Content-Type: application/json" \
-H "Authorization: [Authorization key]" \
-d '{
"from": {"address": "yourname@yourdomain.com"},
"to": [{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}
}],
"subject":"Test Email",
"htmlbody":" Test email sent successfully. "}'
// https://www.npmjs.com/package/zeptomail
// For ES6
import { SendMailClient } from "zeptomail";
// For CommonJS
// var { SendMailClient } = require("zeptomail");
const url = "zeptomail.zoho.com/";
const token = "[Authorization key]";
let client = new SendMailClient({ url, token });
client
.sendMail({
from: {
address: "yourname@yourdomain.com",
name: "noreply"
},
to: [
{
email_address: {
address: "receiver@yourdomain.com",
name: "Receiver"
},
},
],
subject: "Test Email",
htmlbody: " Test email sent successfully.",
})
.then((resp) => console.log("success"))
.catch((error) => console.log("error"));
using System;
using System.Net;
using System.Text;
using System.IO;
using System.Net.Http;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
namespace Rextester {
public class Program {
public static void Main(string[] args) {
System.Net.ServicePointManager.SecurityProtocol =
System.Net.SecurityProtocolType.Tls12;
var baseAddress = "https://zeptomail.zoho.com/v1.1/email";
var http = (HttpWebRequest)WebRequest.Create(new Uri(baseAddress));
http.Accept = "application/json";
http.ContentType = "application/json";
http.Method = "POST";
http.PreAuthenticate = true;
http.Headers.Add("Authorization", "[Authorization key]");
JObject parsedContent = JObject.Parse("{"+
"'from': {'address': 'yourname@yourdomain.com'},"+
"'to': [{'email_address': {"+
"'address': 'receiver@yourdomain.com',"+
"'name': 'Receiver'"+
"}}],"+
"'subject':'Test Email',"+
"'htmlbody':' Test email sent successfully.'"+
"}");
Console.WriteLine(parsedContent.ToString());
ASCIIEncoding encoding = new ASCIIEncoding();
Byte[] bytes = encoding.GetBytes(parsedContent.ToString());
Stream newStream = http.GetRequestStream();
newStream.Write(bytes, 0, bytes.Length);
newStream.Close();
var response = http.GetResponse();
var stream = response.GetResponseStream();
var sr = new StreamReader(stream);
var content = sr.ReadToEnd();
Console.WriteLine(content);
}
}
}
import requests
url = "https://zeptomail.zoho.com/v1.1/email"
payload = """{
"from": {
"address": "yourname@yourdomain.com"
},
"to": [{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}}],
"subject":"Test Email",
"htmlbody":"Test email sent successfully."
}"""
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "[Authorization key]",
}
response = requests.request("POST",url,data=payload,headers=headers)
print(response.text)
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://zeptomail.zoho.com/v1.1/email",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_SSLVERSION => CURL_SSLVERSION_TLSv1_2,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => '{
"from": { "address": "yourname@yourdomain.com"},
"to": [
{
"email_address": {
"address": "receiver@yourdomain.com",
"name": "Receiver"
}
}
],
"subject":"Test Email",
"htmlbody":" Test email sent successfully. ",
}',
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"authorization: [Authorization key]",
"cache-control: no-cache",
"content-type: application/json",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
?>
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class JavaSendapi {
public static void main(String[] args) throws Exception {
String postUrl = "https://zeptomail.zoho.com/v1.1/email";
BufferedReader br = null;
HttpURLConnection conn = null;
String output = null;
StringBuffer sb = new StringBuffer();
try {
URL url = new URL(postUrl);
conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Accept", "application/json");
conn.setRequestProperty("Authorization", "[Authorization key]");
JSONObject object = new JSONObject("{\n" +
" \"from\": {\n" +
" \"address\": \"yourname@yourdomain.com\"\n" +
" },\n" +
" \"to\": [\n" +
" {\n" +
" \"email_address\": {\n" +
" \"address\": \"receiver@yourdomain.com\",\n" +
" \"name\": \"Receiver\"\n" +
" }\n" +
" }\n" +
" ],\n" +
" \"subject\": \"Test Email\",\n" +
" \"htmlbody\": \" Test email sent successfully.\"\n" +
"}");
OutputStream os = conn.getOutputStream();
os.write(object.toString().getBytes());
os.flush();
br = new BufferedReader(
new InputStreamReader((conn.getInputStream()))
);
while ((output = br.readLine()) != null) {
sb.append(output);
}
System.out.println(sb.toString());
} catch (Exception e) {
br = new BufferedReader(
new InputStreamReader((conn.getErrorStream()))
);
while ((output = br.readLine()) != null) {
sb.append(output);
}
System.out.println(sb.toString());
} finally {
try {
if (br != null) {
br.close();
}
} catch (Exception e) {
e.printStackTrace();
}
try {
if (conn != null) {
conn.disconnect();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Benefits of ZeptoMail's transactional email API
Transactional Email
Good deliverability
Power your transactional emails using a trusted and reliable email service. ZeptoMail's exclusive focus on transactional emails gets you great email deliverability.
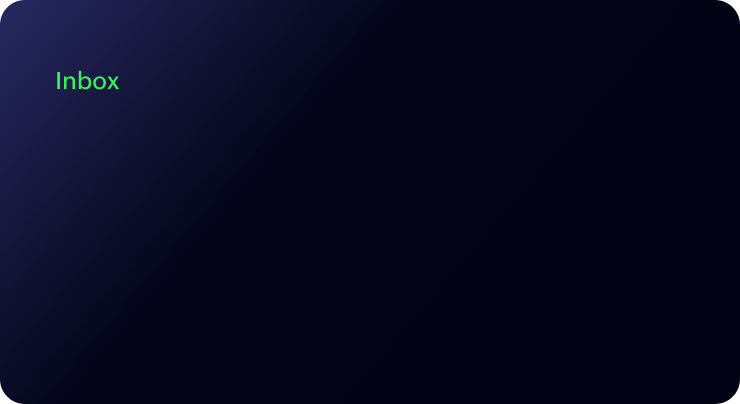
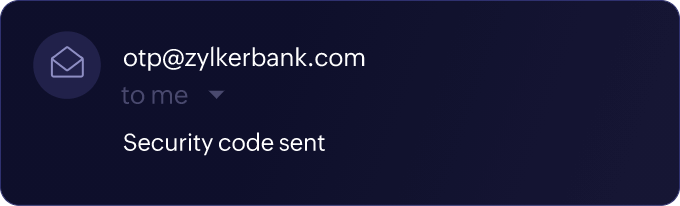
Great customer experience
ZeptoMail delivers emails on time straight into the recipient's inbox. With our email APIs, your users won't be left waiting for their transactional emails or searching for them in the spam folder.
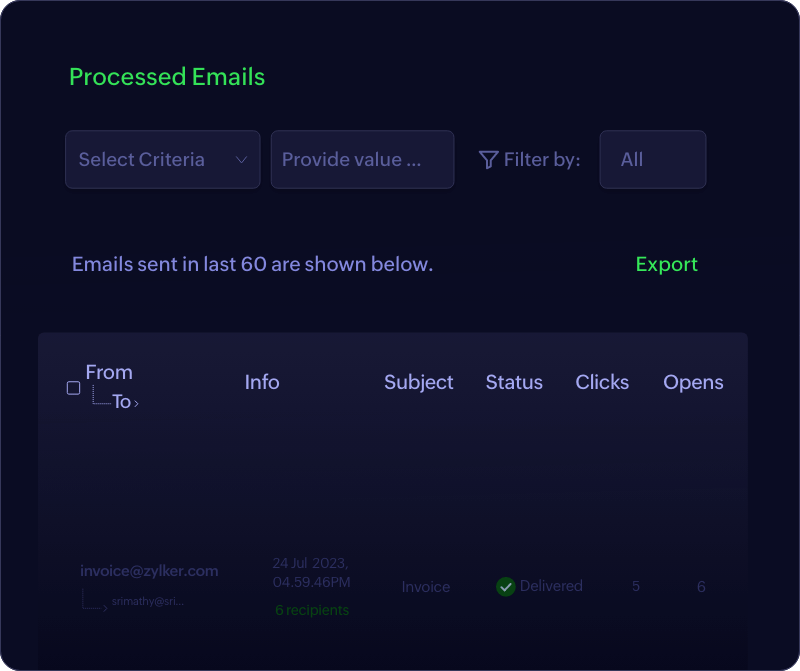

Monitoring capabilities
Sending your transactional emails using ZeptoMail API allows you to view a detailed log of every email that was processed by the server.
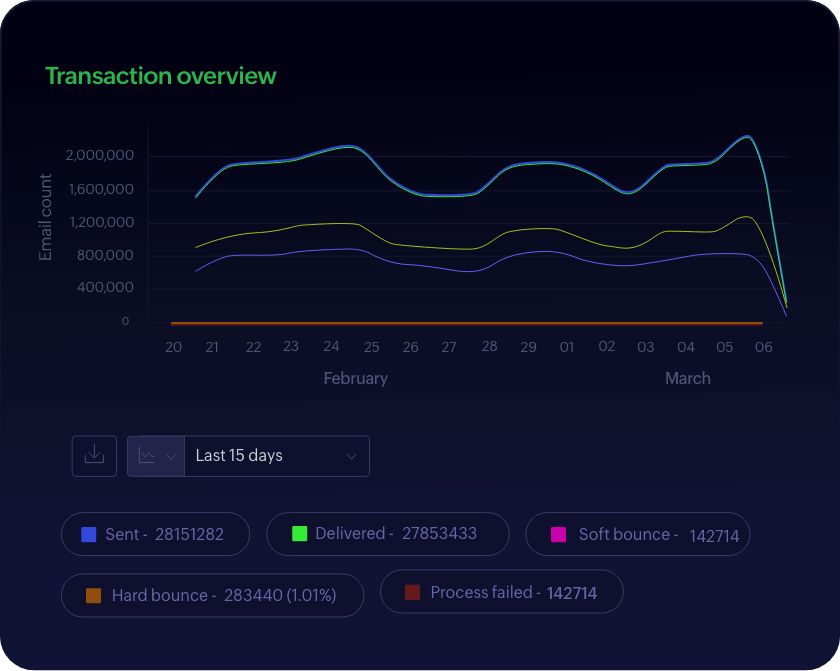
Email analytics
You can enable email tracking in ZeptoMail so you can monitor email performance through recipient activities such as bounces, opens, and clicks.
Detailed documentation
Developer-friendly documentation makes getting started with our APIs easy. The user guide gives step-by-step instructions on how to monitor your emails.
Extensive support
A team of knowledgeable technical experts are available around the clock to help you with any questions or troubleshooting any issues.