Scripting NodeJS Functions
Table of Contents
You can now script your functions in NodeJS and associate them with workflows, schedules, buttons, blueprints, related lists, widgets, or have them serve as standalone functions.
This feature in CRM gives you the freedom and ease of scripting in the language that you are comfortable with.
Inline Edit
Go to Setup > Developer Space > Functions > +New Function. The Create New Function pop up opens.
Enter the following details.
The name of your function.
The display name of your function. This name is what you will see under the My Functions tab.
Description for your function.
Choose the category as Standalone, Button, Automation, Related List, or Schedules from the list.
Select NodeJS from the Language drop-down.
Select the Inline Edit radio button and click Next.
The built-in editor for Zoho CRM opens. This editor lets you write the function code from scratch within the product itself, and takes care of scripting and deployment.
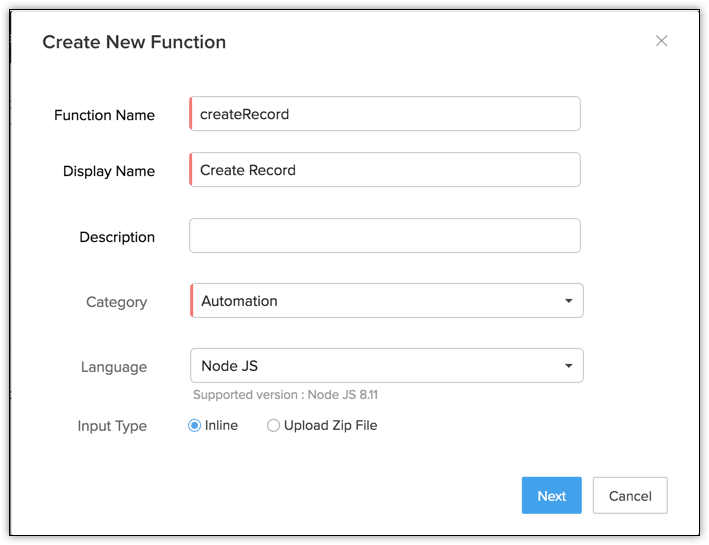
config.json
Zoho CRM automatically packages your NodeJS function and creates the config.json and library files. Specify the values of the following keys.

path: The absolute path to your .js file that contains the function code.
action: The action that your code performs. This is also the name of the main class in your .js file.
connector: The name of the connection that your function invokes, if any.
Default Parameters Object
The crmAPIRequest object is the hidden parameter that contains information about your organization, users, variables, and records. This object has four default keys—org, users, variables, and record. Use these parameters in your code as depicted below, to retrieve information easily while performing your business logic.
module.exports = async function( context, basicIO )
{
/* 1)entity_object 2)user_object 3)organization_object 4)variables_object 5)request_object are the default parameters which contains entity information, user information,
organization information, variables inforamtion and request information in it respectively.
2) For Button Mass action and RL Button, ids list will be available in basicIO object. you can get by basicIO.getParameter("ids") & basicIO.getParameter("related_entity_ids") respectively
*/
// To get the Entity Information
var entityObject = basicIO.getParameter("entity_object");
// To get the Organization Information
var organizationObject = basicIO.getParameter("organization_object");
//To get the User Information
var userObject = basicIO.getParameter("user_object");
// To get the CRM Variables Information
var variablesObject = basicIO.getParameter("variables_object");
// To get the Request Information
var requestObject = basicIO.getParameter("request_object");
basicIO.write("Function Executed Successfully");
context.log.INFO("log data");
context.close();
}
variables_object returns the current user's details in the response. If the function is configured as a REST API or associated to a schedule, this object returns the details of the primary user of your organization.
variables_object returns the variables configured for your organization.
organization_object returns the information about your organization.
entity_object returns the information about the record whose ID and module name you specify in the input.
context object is the deluge equivalent of the "info" statement. You can use this to debug the response and access the response logs using "context.log".
basicIO object is the NodeJS object that is available by default, and contains the hidden parameter "crmAPIRequest".
Use "basicIO.write()" to display a value in the response. This is equivalent to the "return" statement in Deluge.
When you Save & Execute the above code, the Input Values pop up opens. You can specify the values of your arguments either as a JSON body or as key-value pairs.
When you specify the input keys in the Input Values pop up, do not use the default keys of the crmAPIRequest object. For example, if you specify the input key-value pair as "variables":"Patricia", instead of returning the variables in your organization, the response will contain the input message that you provided, that is, system overrides the action that the crmAPIRequestObj performs.
To pass the arguments of your function in the body, use the below code.
module.exports = async function( context, basicIO )
{
// To get the Request Information
var requestObject = basicIO.getParameter("request_object");
var requestBody = JSON.parse(requestObject);
var body = JSON.parse(requestBody.body);
basicIO.write(JSON.stringify(body.param1));
basicIO.write(JSON.stringify(body.param2));
basicIO.write("---------------------------------------------------");
context.log.INFO("log data");
context.close();
}
The input body must be a JSON object with the values for the arguments used in the code.
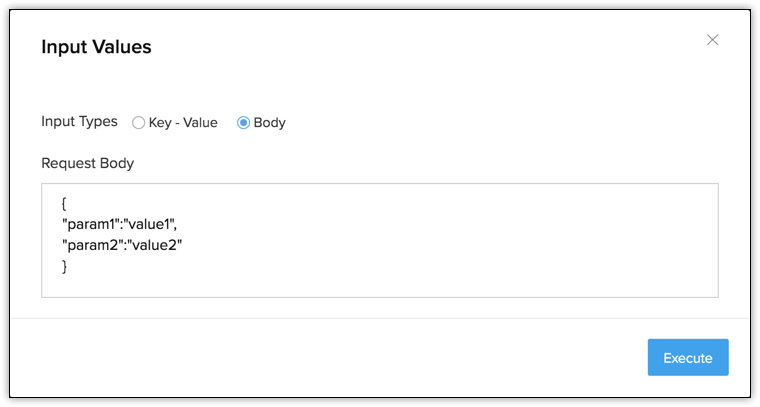
To pass the arguments of your function as key-value pairs, use the below code.
module.exports = async function( context, basicIO )
{
// To get the Request Information
var requestObject = basicIO.getParameter("request_object");
var requestParams = JSON.parse(requestObject);
basicIO.write(JSON.stringify(requestParams.keyValue1));
context.log.INFO("log data");
context.close();
}
The input values to the arguments used in the function must be key-value pairs.
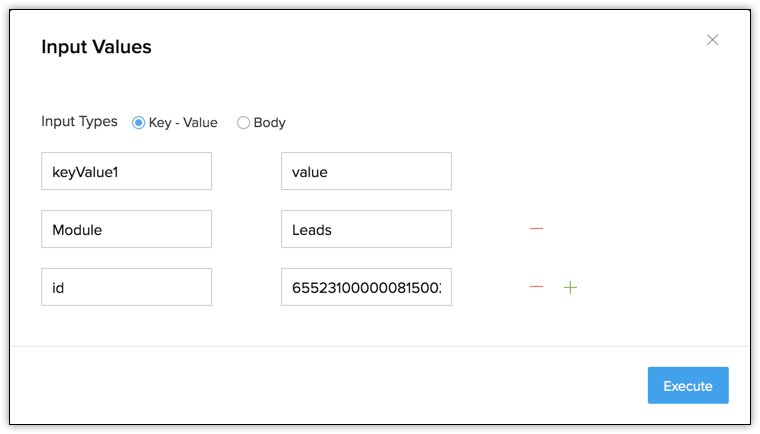
node_modules
The node_modules contains inbuilt functions present in NodeJS library classes, to help developers perform tasks easily. You can upload files to the library of a NodeJS function.
Right click "node_modules" and select Upload Files. The File Upload dialog box opens.
Click Choose File and select the JS libraries you want to upload.
Click Upload. The files will get added to the library.
You can upload only JS libraries to node_modules.
The size of all the files(in total) that you want to upload must not exceed 10MB.
Sample: Create a record
module.exports = async function (context, basicIO) {
var connector = context.getConnection("znew");
let leadinfo = {
"data": [
{
"Company": "Zylker",
"Last_Name": "Boyle",
"Phone": "12345",
"Email": "p.boyle@abc.com"
}
]
};
var connRes = await connector.makeRequestSync({
url: 'https://crm.zoho.com/crm/v2/Leads',
method: "POST"
}, JSON.stringify(leadinfo));
context.log.INFO(connRes.statusCode);
connRes.on("data", function (data) {
basicIO.write(String(data));
})
var promiseFunction = function () {
return new Promise(function (resolve, reject) {
connRes.on("error", function (error) {
basicIO.write(String(error));
resolve("Error");
});
connRes.on("end", resolve);
});
}
}
Sample: Update a record
module.exports = async function (context, basicIO) {
var connector = context.getConnection("znew");
let leadinfo = {
"data": [
{
"Company": "Zylker",
"Last_Name": "Boyle",
"Phone": "12345",
"Email": "p.boyle@abc.com"
}
]
};
var connRes = await connector.makeRequestSync({
url: 'https://crm.zoho.com/crm/v2/Leads/4848534000000314422',
method: "PUT"
}, JSON.stringify(leadinfo));
context.log.INFO(connRes.statusCode);
connRes.on("data", function (data) {
basicIO.write(String(data));
})
var promiseFunction = function () {
return new Promise(function (resolve, reject) {
connRes.on("error", function (error) {
basicIO.write(String(error));
resolve("Error");
});
connRes.on("end", resolve);
});
}
}
Sample: Get a record
module.exports = async function (context, basicIO) {
var connector = context.getConnection("znew");
var connRes = await connector.makeRequestSync({
url: 'https://crm.zoho.com/crm/v2/Leads/4848534000000314419',
method: "GET"
});
context.log.INFO(connRes.statusCode);
connRes.on("data", function (data) {
basicIO.write(String(data));
})
var promiseFunction = function () {
return new Promise(function (resolve, reject) {
connRes.on("error", function (error) {
basicIO.write(String(error));
resolve("Error");
});
connRes.on("end", resolve);
});
}
}
Sample: Search for a record
module.exports = async function (context, basicIO) {
var connector = context.getConnection("znew");
var connRes = await connector.makeRequestSync({
url: 'https://crm.zoho.com/crm/v2/Leads/search?criteria=Last_Name:equals:Boyle',
method: "GET"
});
context.log.INFO(connRes.statusCode);
connRes.on("data", function (data) {
basicIO.write(String(data));
})
var promiseFunction = function () {
return new Promise(function (resolve, reject) {
connRes.on("error", function (error) {
basicIO.write(String(error));
resolve("Error");
});
connRes.on("end", resolve);
});
}
}
Upload a ZIP File
The other way is to script the function in any programming language editor and upload the folder to CRM. You can also bundle external libraries and upload them.
To bundle the external libraries in your NodeJS function
Create a folder containing config.json, node_modules, and the JS file.
Install the external libraries in the node_modules folder.
ZIP the folder and create a function with the ZIP file.
The NodeJS editor uses the NodeJS 8.11. You must use the libraries corresponding to this version of NodeJS.
You can add the external libraries as a zip only when you create a NodeJS function. You cannot add them to the existing functions.
You can upload only one zip file per function.
The maximum allowed size of the zip file is 10MB.
The Function name, the ZIP file name and the main JS file name should be the same, or the system will throw an error. Please note that naming is case-sensitive.
Once the file is uploaded, the in-product editor opens, where you can make modifications to the code.